Logical Operators
So far, we've used comparison operators to filter the data. But in real-world scenarios, we often have to retrieve the data using several conditions at once. For example, in the case of e-commerce platforms, users often search for something like: Get shoes from the Puma brand, which have ratings greater than 4.0 and price less than 5000.
With logical operators, we can perform queries based on multiple conditions. Let's learn how with the following database.
Database The database contains a
AND, OR, NOT
Operator | Description |
---|---|
AND | Used to fetch rows that satisfy two or more conditions. |
OR | Used to fetch rows that satisfy at least one of the given conditions. |
NOT | Used to negate a condition in the WHERE clause. |
Syntax
Examples
- Get all the details of the products whose
- categoryis "Clothing" and
- priceless than or equal to 1000 from theproducttable.
Output
name | category | price | brand | rating |
---|---|---|---|---|
Blue Shirt | Clothing | 750 | Denim | 3.8 |
Blue Jeans | Clothing | 800 | Puma | 3.6 |
Black Jeans | Clothing | 750 | Denim | 4.5 |
... | ... | ... | ... | ... |
- Ignore all the products withnamecontaining "Cake" from the list of products.
Output
name | category | price | brand | rating |
---|---|---|---|---|
Blue Shirt | Clothing | 750 | Denim | 3.8 |
Blue Jeans | Clothing | 800 | Puma | 3.6 |
Black Jeans | Clothing | 750 | Denim | 4.5 |
--- | --- | --- | --- | --- |
Try it Yourself!
- Fetch all the products withpriceless than 20000 andbrandis "Apple".
- Fetch all the products withratinggreater than 4.0 orbrandis "Britannia".
- Ignore all the products withcategorycontaining "Food" inproducttable.
Multiple Logical Operators
We can also use the combinations of logical operators to combine two or more conditions. These compound conditions enable us to fine-tune the data retrieval requirements.
Precedence
- When a query has multiple operators, operator precedence determines the sequence of operations.
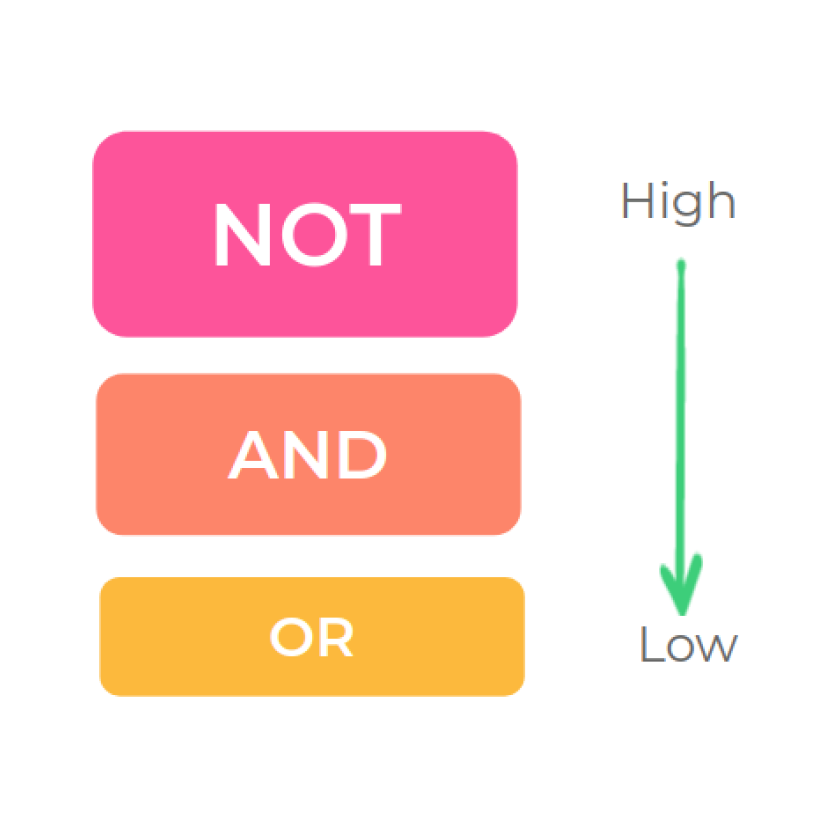
Order of precedence:
- NOT
- AND
- OR
Example
Fetch the products that belong to
- Redmi brandandratinggreater than 4 or
the products from OnePlus
brandSQLIn the above query,
ANDhas the precedence overOR. So, the above query is equivalent to:
Try it Yourself!
- Fetch all the products from "Clothing" category whosenamedoes not contain "Jeans".
- Fetch all the products from "Puma" and "Denim" brands excluding the products withnamecontaining "Shirts".
- Fetch all the products withpriceless than 100 or the products from "Food" category excluding the ones withnamecontaining "Chocolate"