1. What are logical operators?
The logical operators are used to perform logical operations on Boolean values.
Gives
Following are the logical operators
- and
- or
- not
Logical AND Operator
Gives
Code
Output
Example
Code
Step by Step Explanation
Output
Logical OR Operator
Gives
Code
Output
Example
Code
Step by Step Explanation
Output
Logical NOT Operator
Gives the opposite value of the given boolean.
Code
Output
Example
Code
Step by Step Explanation
Output
2. What are conditional statements?
The Conditional Statement allows you to execute a block of code based on a condition.
If statement
The Conditional Statement allows you to execute a block of code only when a specific condition is
code
Output
If-Else statement
When If-Else conditional statement is used, the Else block of code executes if the condition is
Using If-Else
Code
Input
Output
3. What are Loops?
Loops allow us to execute a block of code several times.
The loops in Python are:
- While Loop
- For Loop
4. What is the use of while loop?
While loop allows us to execute a block of code several times as long as the condition is
Example
The following code snippet prints the next three consecutive numbers after a given number.
Code
Input
Output
5. How to create a do-while loop in python?
Generally, in other programming languages, we will have another loop called the
In
The
Example: do-while loop in c language
code
output
If the condition checked evaluates to True, the loop continues. For the cases where you would want your code to run at least one time then the
do-while loop in python:
In Python, we can create a
Code
Output
6. What is the use of for loop?
The
Examples of sequences:
- The sequence of Characters (string)
- The sequence of numbers, etc.
Code
Output
7. What are range() and xrange() functions?
range():
The
Syntax:
Code
Output
Range with Start and End
Syntax:
Generates a sequence of numbers starting from
Code
Output
xrange():
The
Code
Output
Note: The
8. When does an infinite loop occurs?
An infinite loop occurs when the condition always evaluates to
Example
Code
In the above code the while loop will run infinite times as the condition always evaluates to
9. What are Nested Loops?
An inner loop within the repeating block of an outer loop is called Nested Loop.
The Inner Loop will be executed one time for each iteration of the Outer Loop.
Code
Output
10. What is a break statement?/ How to exit from a loop?
Break statement makes the program exit a loop early.
Using Break
Generally,
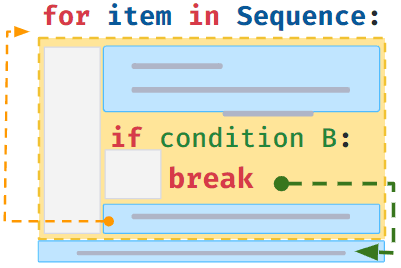
In the below example, when the variable
Code
Output
Break in Nested Loop
The
Code
Output
11. What is continue in loops?
The
Using continue
Generally,
In the below example, when the variable
Code
Output
12. What is pass in Python?
The
Generally it used when we have to test the code before writing the complete code.
Empty Loops
We can use