1. Reconciliation
1.1 Virtual DOM
A Virtual DOM is a JS object, which is the virtual representation of an HTML DOM.
Whenever new elements are added to the UI, a virtual DOM is created.
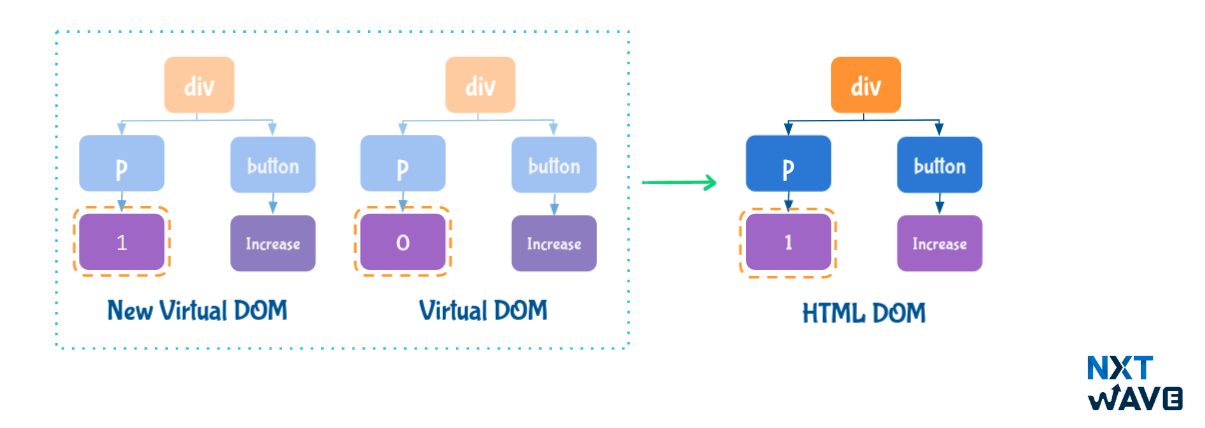
React compares new virtual DOM with current virtual DOM, and the difference will be efficiently updated to HTML DOM. So, the Virtual DOM helps to render the UI more performantly.
React only updates what's necessary. This process is known as Reconciliation.
2. React Batch Updating
React combines multiple
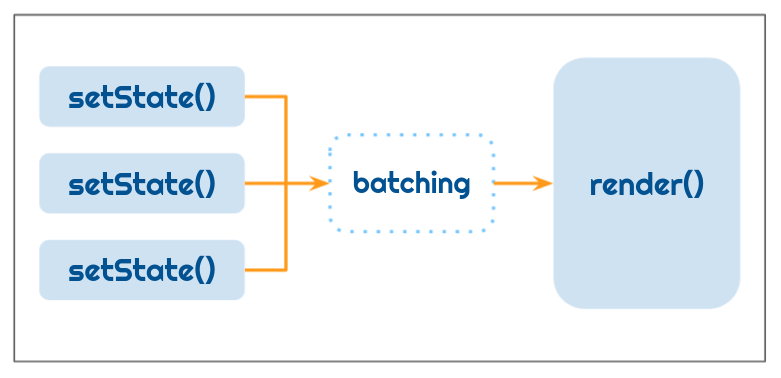
Example:
In the above example, the
3. setState() Syntax
3.1 Object Syntax
Object Syntax is used while updating the state to a value.
3.2 Callback Syntax
Callback Syntax is used while updating the state to a value that is computed based on the previous state.
3.3 Object Syntax vs Callback Syntax
Object Syntax:
When the HTML
When the Object Syntax is used,
Callback Syntax:
When the HTML
The
4. Children Prop
The children is a special prop that is automatically passed to every React Component.
The JSX Element/Text included in between the opening and closing tags are considered children.
4.1 Passing Text as Children
File: src/components/Post/index.js
4.2 Accessing Children
File: src/components/SocialButton/index.js
5. Controlled vs Uncontrolled Input
In React, the Input Element
- Controlled Input
- Uncontrolled Input
5.1 Controlled Input
If the Input Element
It is the React Suggested way to handle the Input Element value.
Example:
5.2 Uncontrolled Input
If the Input Element
Example:
6. Props vs State
- The props and state are plain JS objects.
- The props and state changes trigger the render method.
Props | State |
---|---|
Props get passed to the component, similar to function parameters | State is created and managed within the component, similar to a variable declared within the function |
Props are used to pass data and event handlers down to the child components | State is used to store the component's data that changes over time |
Props are immutable. A component cannot change the props | State should be immutable |
7. State should be minimal
We need the state to make the applications interactive.
To build the application correctly, you first need to think of the minimal set of the state that the application needs.
Let's take an example application, Projects.
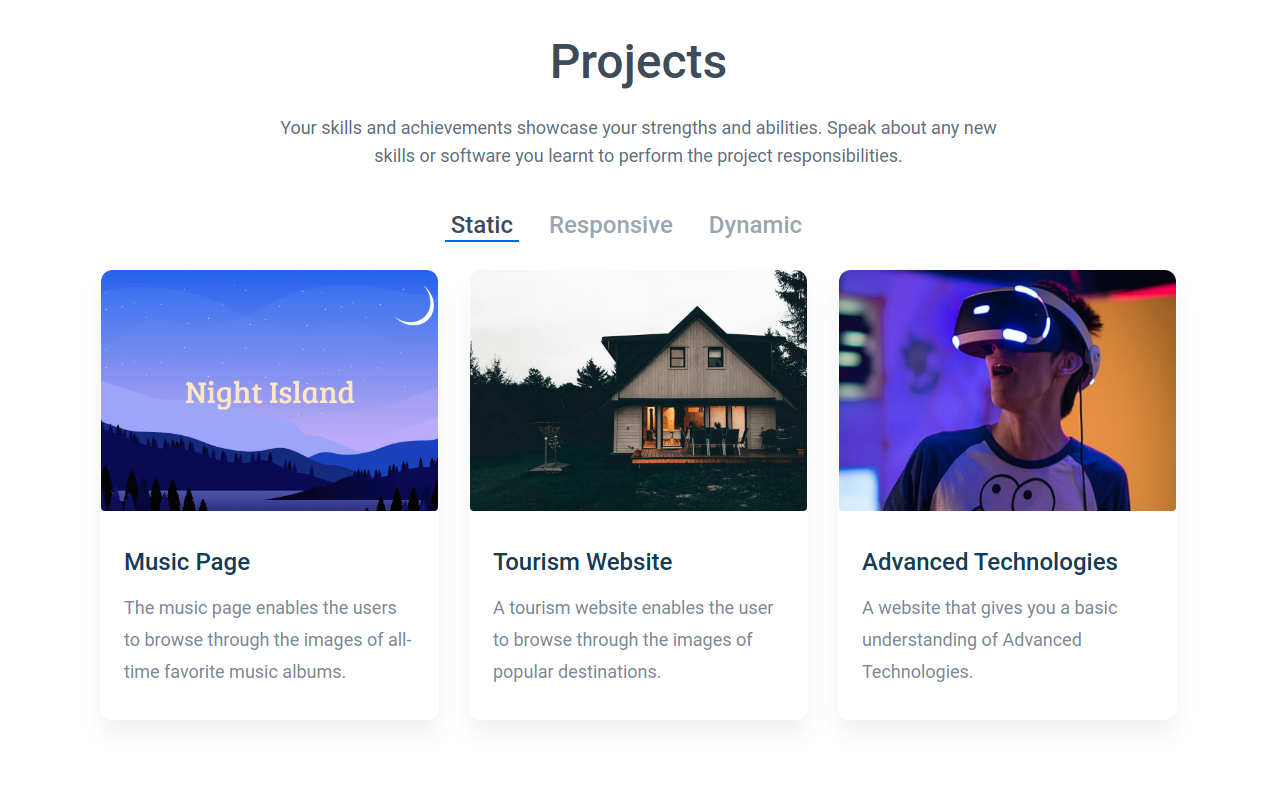
Think of all the pieces of data in the Projects Application. We have:
- The original list of projects
- The active tab item
- The text decoration of the text - Underline
- The color of the text
- The filtered list of projects
Let's go through each one and figure out which one is state. Ask three questions about each piece of data:
- Is it passed in from a parent via props? If so, it probably isn't state.
- Does it remain unchanged over time? If so, it probably isn't state.
- Can it be computed based on any other state or props in the component? If so, it isn't state.
Let's figure out which one is state in our application:
Data | Is State? | Reason |
---|---|---|
The original list of projects | No | It is passed as props |
The active tab item | Yes | It changes over time and can't be computed from anything |
The filtered list of projects | No | It can be computed by combining the original list of projects with the active tab item |
The text decoration of the text - Underline | No | It can be derived from the active tab item |
The The color of the text | No | It can be derived from the active tab item |
So finally, the state is:
- The active tab item
8. Keys
Keys help React identify which items have been changed, added, or removed.
Keys should be given to the elements inside the array to give a stable identity.
8.1 Keys in Lists
When the state of a component changes, React updates the virtual DOM tree. Once the virtual DOM has been updated, React then compares the new virtual DOM with the current virtual DOM.
Once React knows which virtual DOM objects have changed, then React updates only those objects, in the HTML DOM.
React compares the virtual DOMs if there is a change in the node, node type, or the attributes passed to the node. But there is a problematic case by only looking at the node or it's attributes. i.e. Lists.
Lists will have the same node types and attributes. Hence the list items can't be uniquely identified if they have been changed, added, or removed.
Example-1:
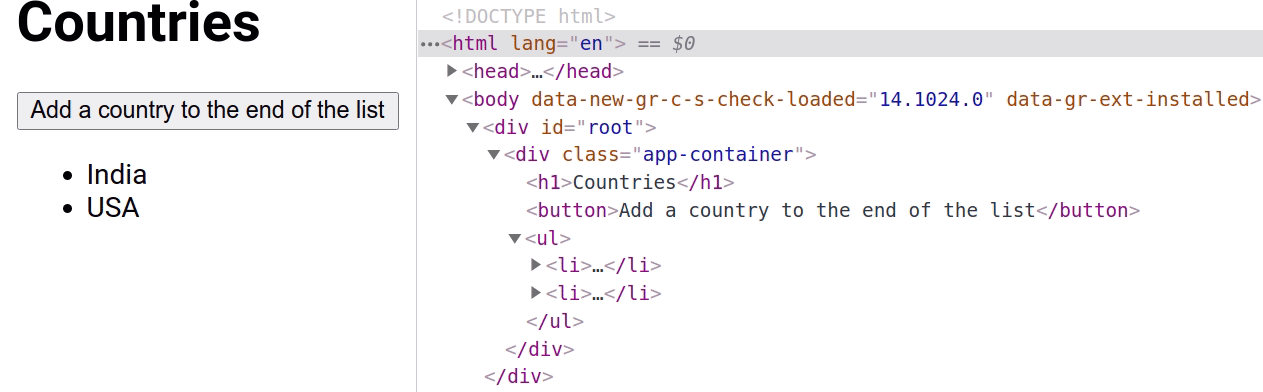
When a new list item is added to the end of the list,
- React creates a new virtual DOM with the three list items.
- React compares the first two list items in the current virtual DOM and the new virtual DOM.
- Since the two list items in both the previous and current virtual DOMs are matched, React creates only the last list item at the end in the HTML DOM.
Example-2:
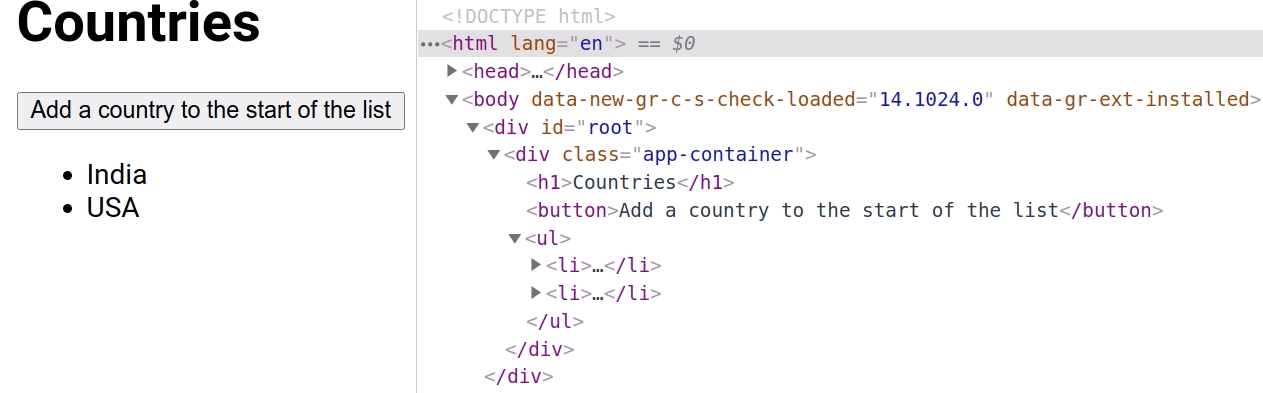
When a new list item is added to the start of the list,
- React creates a new virtual DOM with the three list items.
- React compares the first list item in the current virtual DOM and the new virtual DOM.
- Since the first list item in both the previous and current virtual DOMs is different, React treats as the whole list is changed in the current virtual DOM.
- React creates all the three list items in the HTML DOM.
So, React will recreate every element, instead of reusing the two elements that remained the same between the previous and current virtual DOMs. It leads to bad performance.
That's where keys are important. Using keys, every item in a list will have a unique identifier (ID).
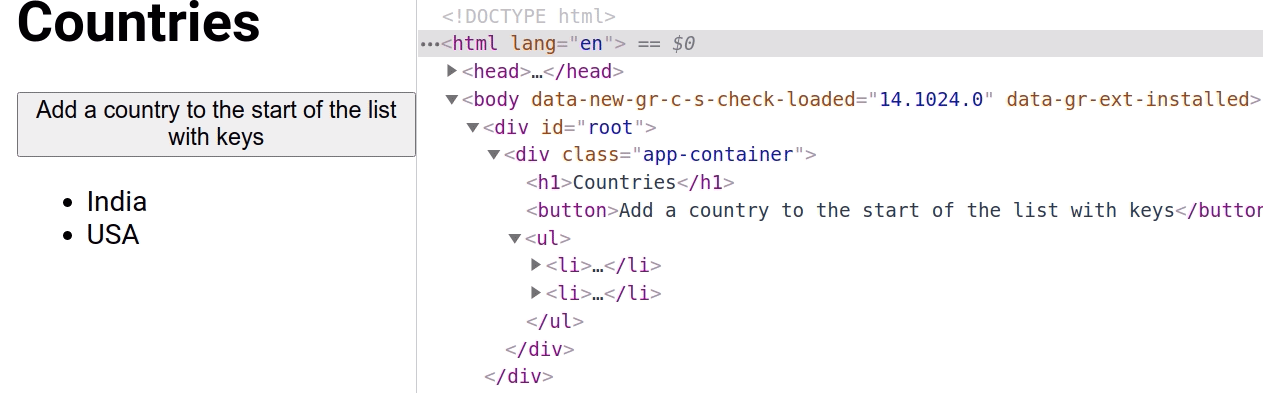
So, React can easily detect what needs to be changed or not, re-rendering only the ones with changes.