Object
In general, anything that can be assigned to a variable in Python is referred to as an object.
Strings, Integers, Floats, Lists, Functions, Module etc. are all objects.
Identity of an Object
Whenever an object is created in Python, it will be given a unique identifier (id).This unique id can be different for each time you run the program.
Every object that you use in a Python Program will be stored in Computer Memory
The unique id will be related to the location where the object is stored in the Computer Memory.
Name of an Object
Name or Identifier is simply a name given to an object.
Namespaces
A namespace is a collection of currently defined names along with information about the object that the name references.
It ensures that names are unique and won’t lead to any conflict.
Namespaces allow us to have the same name referring different things in different namespaces.
Code
Output
Types of namespaces
As Python executes a program, it creates namespaces as necessary and forgets them when they are no longer needed.
Different namespaces are:
Built-in
Global
Local
Built-in Namespace
Created when we start executing a Python program and exists as long as the program is running.
This is the reason that built-in functions like id(), print() etc. are always available to us from any part of the program.
Global Namespace
This namespace includes all names defined directly in a module (outside of all functions).
It is created when the module is loaded, and it lasts until the program ends.
Local Namespace
Modules can have various
A new local namespace is created when a function is called, which lasts until the function returns.
Scope of a Name
The scope of a name is the region of a program in which that name has meaning.
Python searches for a name from the inside out, looking in the
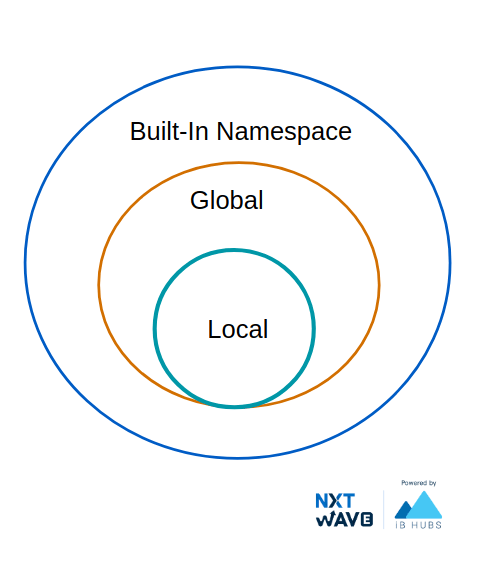
Global variables
In Python, a variable defined outside of all functions is known as a global variable.
This variable name will be part of Global Namespace.
Example 1
Code
Output
Example 2
Code
Output
Local Variables
In Python, a variable defined inside a function is a local variable.
This variable name will be part of the Local Namespace which will be created when the function is called and lasts until the function returns.
Code
Output
As,