Standard Library
Built-in Functions
Built-in functions are Readily available for reuse.
Some of the built Functions are
- print()
- max()
- min()
- len()and many more..
Standard Library
Python provides several such useful values (constants), classes and functions.
This collection of predefined utilities is referred as the Python Standard Library
All these functionalities are organized into different modules.
- In Python context, any file containing a Python code is called a module
- These modules are further organized into folders known as packages
Different modules are:
collections
random
datetime
math and many more..
Working with Standard Library
To use a functionality defined in a module we need to import that module in our program.
Math Module
math module provides us to access some common math functions and constants.
Code
Output
Importing module
Importing a module and giving it a new name (aliasing)
Code
Output
Importing from a Module
We can import just a specific definition from a module.
Code
Output
Aliasing Imports
We can also import a specific definition from a module and alias it
Code
Output
Random module
Randomness is useful in whenever uncertainty is required.
For example: Rolling a dice, flipping a coin, etc.,
Randint
Code
Output
Choice
Code
Output
To know more about Python Standard Library, go through the authentic python documentation - https://docs.python.org/3/library/
Map, Filter and Reduce
We worked with different sequences (list, tuples, etc.)
To simplify working with sequences we can use
Map
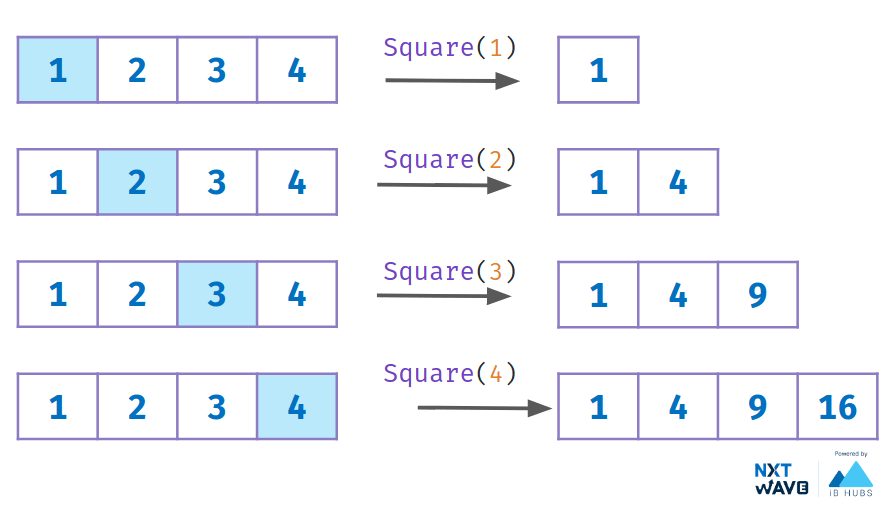
Example - 1
Code
Output
Example - 2
Code
Input
Output
Filter
The function should return True/False